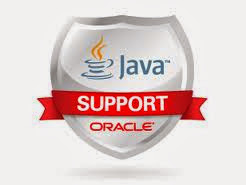
Hello friends I have recently worked on a project on Java where I had to create a
Download Manager for Java using Swings. After a lots of effort I finally succeeded to create the Download manager. Here I am Posting the code that I have used for creating the download manager.
Download manager for Java
Download Source Code
Download.java
import java.io.*;
import java.util.*;
import java.net.*;
class Download extends Observable implements Runnable {
private static final int MAX=1024;
public static final String STATUSES[]={"Downloading","Paused","Complete","Cancelled","Error"};
public static final int DOWNLOADING=0;
public static final int PAUSED=1;
public static final int COMPLETE=2;
public static final int CANCELLED=3;
public static final int ERROR=4;
private URL url;
private int size;
private int downloaded;
private int status;
public Download(URL url) {
this.url=url;
size=-1;
downloaded=0;
status=DOWNLOADING;
}
public String getUrl() {
return url.toString();
}
public int getSize() {
return size;
}
public float getProgress() {
return ((float)downloaded/size)*100;
}
public int getStatus() {
return status;
}
public void pause() {
status=PAUSED;
stateChanged();
}
public void resume() {
status=DOWNLOADING;
stateChanged();
download();
}
public void cancel() {
status=CANCELLED;
stateChanged();
}
private void error() {
status=ERROR;
stateChanged();
}
private void download() {
Thread thread=new Thread(this);
thread.start();
}
private String getFileName(URL url) {
String fileName=url.getFile();
return fileName.substring(fileName.lastIndexOf('/')+1);
}
public void run() {
RandomAccessFile file=null;
InputStream stream=null;
try {
HttpURLConnection connection=(HttpURLConnection) url.openConnection();
connection.setRequestProperty("Range","byte="+downloaded+"-");
connection.connect();
if(connection.getResponseCode()/100!=2) {
error();
}
int contentLength=connection.getContentLength();
if(size==-1) {
size=contentLength;
stateChanged();
}
file= new RandomAccessFile(getFileName(url),"rw");
file.seek(downloaded);
stream=connection.getInputStream();
while(status==DOWNLOADING) {
byte buffer[];
if(size-downloaded>MAX) {
buffer=new byte[MAX];
}
else {
buffer=new byte[size-downloaded];
}
int read=stream.read(buffer);
if(read==-1) break;
file.write(buffer,0,read);
downloaded+=read;
stateChanged();
}
if(status==DOWNLOADING) {
status=COMPLETE;
stateChanged();
}
}
catch(Exception e) {
error();
}
finally {
if(file!=null) {
try {
file.close();
}
catch(Exception e){} }
}
if(stream!=null) {
try {
stream.close();
}
catch(Exception e){} }
}
private void stateChanged() {
setChanged();
notifyObservers();
}
}
DownloadManager.java
import java.awt.*;
import java.awt.event.*;
import java.net.*;
import java.util.*;
import javax.swing.*;
import javax.swing.event.*;
public class DownloadManager extends JFrame implements Observer {
private JTextField addTextField;
private DownloadsTableModel tableModel;
private JTable table;
private JButton pauseButton,resumeButton;
private JButton cancelButton,clearButton;
private Download selectedDownload;
private boolean clearing;
public DownloadManager()
{
setTitle("My Download Manager");
setSize(640,640);
addWindowListener(new WindowAdapter()
{
public void WindowClosing(WindowEvent e)
{
actionExit();
}
});
JMenuBar menuBar=new JMenuBar();
JMenu fileMenu=new JMenu("File");
fileMenu.setMnemonic(KeyEvent.VK_F);
JMenuItem fileExitMenuItem=new JMenuItem("Exit",KeyEvent.VK_X);
fileExitMenuItem.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
actionExit();
}
});
fileMenu.add(fileExitMenuItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
JPanel addPanel=new JPanel();
addTextField=new JTextField(30);
addPanel.add(addTextField);
JButton addButton=new JButton("Add DOwnload");
addButton.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
actionAdd();
}
});
addPanel.add(addButton);
tableModel=new DownloadsTableModel();
table=new JTable(tableModel);
table.getSelectionModel().addListSelectionListener(new ListSelectionListener()
{
public void valueChanged(ListSelectionEvent e)
{
tableSelectionChanged();
}
});
table.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
ProgressRenderer renderer=new ProgressRenderer(0,100);
renderer.setStringPainted(true);
//table.setDefaultRenderer(JProgressBar.class,renderer);
table.setRowHeight((int) renderer.getPreferredSize().getHeight());
JPanel downloadsPanel=new JPanel();
downloadsPanel.setBorder(BorderFactory.createTitledBorder("Downloads"));
downloadsPanel.setLayout(new BorderLayout());
downloadsPanel.add(new JScrollPane(table), BorderLayout.CENTER);
JPanel buttonsPanel=new JPanel();
pauseButton=new JButton("Pause");
pauseButton.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
actionPause();
}
});
pauseButton.setEnabled(false);
buttonsPanel.add(pauseButton);
resumeButton=new JButton("Resume");
resumeButton.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
actionResume();
}
});
resumeButton.setEnabled(false);
buttonsPanel.add(resumeButton);
cancelButton=new JButton("Cancel");
cancelButton.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
actionCancel();
}
});
cancelButton.setEnabled(false);
buttonsPanel.add(cancelButton);
clearButton=new JButton("Clear");
clearButton.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
actionClear();
}
});
clearButton.setEnabled(false);
buttonsPanel.add(clearButton);
getContentPane().setLayout(new BorderLayout());
getContentPane().add(addPanel,BorderLayout.NORTH);
getContentPane().add(downloadsPanel,BorderLayout.CENTER);
getContentPane().add(buttonsPanel,BorderLayout.SOUTH);
}
private void actionExit()
{
System.exit(0);
}
private void actionAdd()
{
URL verifiedUrl=verifyUrl(addTextField.getText());
if(verifiedUrl!=null)
{
tableModel.addDownload(new Download(verifiedUrl));
addTextField.setText("");
}
else
{
JOptionPane.showMessageDialog(this,"Invalid Download URL", "Error",JOptionPane.ERROR_MESSAGE);
}
}
private URL verifyUrl(String url)
{
if(!url.toLowerCase().startsWith("http://"))
return null;
URL verifiedUrl=null;
try
{
verifiedUrl=new URL(url);
}
catch(Exception e)
{
return null;
}
if(verifiedUrl.getFile().length()<2)
return null;
return verifiedUrl;
}
private void tableSelectionChanged()
{
if(selectedDownload!=null)
{
selectedDownload.deleteObserver(DownloadManager.this);
}
if(!clearing && table.getSelectedRow()>-1)
{
selectedDownload=tableModel.getDownload(table.getSelectedRow());
selectedDownload.addObserver(DownloadManager.this);
updateButtons();
}
}
private void actionPause()
{
selectedDownload.pause();
updateButtons();
}
private void actionResume()
{
selectedDownload.resume();
updateButtons();
}
private void actionCancel()
{
selectedDownload.cancel();
updateButtons();
}
private void actionClear()
{
clearing=true;
tableModel.clearDownload(table.getSelectedRow());
clearing=false;
selectedDownload=null;
updateButtons();
}
private void updateButtons()
{
if(selectedDownload!=null)
{
int status=selectedDownload.getStatus();
switch(status)
{
case Download.DOWNLOADING:pauseButton.setEnabled(true);
resumeButton.setEnabled(false);
cancelButton.setEnabled(true);
clearButton.setEnabled(false);
break;
case Download.PAUSED:pauseButton.setEnabled(false);
resumeButton.setEnabled(true);
cancelButton.setEnabled(true);
clearButton.setEnabled(false);
break;
case Download.ERROR:pauseButton.setEnabled(false);
resumeButton.setEnabled(true);
cancelButton.setEnabled(false);
clearButton.setEnabled(true);
break;
default:pauseButton.setEnabled(false);
resumeButton.setEnabled(false);
cancelButton.setEnabled(false);
clearButton.setEnabled(true);
break;
}
}
else
{
pauseButton.setEnabled(false);
resumeButton.setEnabled(false);
cancelButton.setEnabled(false);
clearButton.setEnabled(false);
}
}
public void update(Observable o,Object arg)
{
if(selectedDownload!=null && selectedDownload.equals(o))
updateButtons();
}
public static void main(String args[])
{
SwingUtilities.invokeLater(new Runnable()
{
public void run()
{
DownloadManager manager=new DownloadManager();
manager.setVisible(true);
}
});
}
}
DownloadsTableModel.java
import java.util.*;
import javax.swing.*;
import javax.swing.table.*;
class DownloadsTableModel extends AbstractTableModel implements Observer {
private static final String[] columnNames={"URL","Size","Progress","Status"};
private static final Class[] columnClasses={String.class,String.class,JProgressBar.class,String.class};
private ArrayList downloadList=new ArrayList();
public void addDownload(Download download)
{
download.addObserver(this);
downloadList.add(download);
fireTableRowsInserted(getRowCount()-1,getRowCount()-1);
}
public Download getDownload(int row)
{
return downloadList.get(row);
}
public void clearDownload(int row)
{
downloadList.remove(row);
fireTableRowsDeleted(row,row);
}
public int getColumnCount()
{
return columnNames.length;
}
public String getColumnName(int col)
{
return columnNames[col];
}
public Class getColumnClass(int col)
{
return columnClasses[col];
}
public int getRowCount()
{
return downloadList.size();
}
public Object getValueAt(int row,int col)
{
Download download=downloadList.get(row);
switch(col)
{
case 0:return download.getUrl();
case 1:int size=download.getSize();
return (size==-1)?"":Integer.toString(size);
case 2:return new Float(download.getProgress());
case 3:return Download.STATUSES[download.getStatus()];
}
return "";
}
public void update(Observable o,Object arg)
{
int index=downloadList.indexOf(o);
fireTableRowsUpdated(index,index);
}
}
ProgressRender.java
import java.awt.*;
import javax.swing.*;
import javax.swing.table.*;
class ProgressRenderer extends JProgressBar {
public ProgressRenderer(int min,int max)
{
super(min,max);
}
public Component getTableCellRendererComponent(JTable table,Object value,boolean isSelected,boolean hasFocus,int row,int column)
{
setValue((int) ((Float) value).floatValue());
return this;
}
}
Above I have used 4 different methods each for performing some specific functionality to make the
Download Manager work. I have also posted the download link for the complete source code above, so download it and have fun. For any issues related to the code or any other doubt feel free to post a comment below.
Sir i got an error in DownloadsTableModel.java
ReplyDeleteat java:16: error: incompatible types
return downloadList.get(row);
and
DownloadsTableModel.java:41: error: incompatible types
Download download=downloadList.get(row);